Python 脚本是一种强大的工具,可用于各种任务,从自动化日常工作到处理复杂的数据操作。以下是一些关于 Python 脚本的高级概念和代码示例。
函数的高级用法
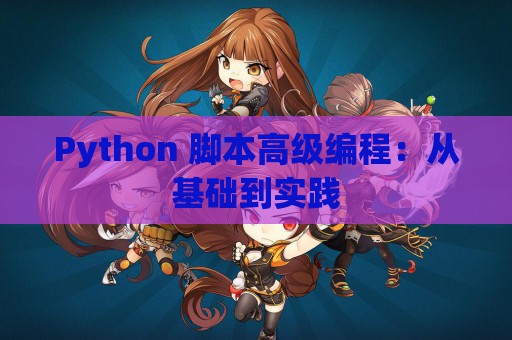
def complex_function(name, age, *args, **kwargs):
print(f"Name: {name}, Age: {age}")
print("Additional arguments:")
for arg in args:
print(arg)
print("Keyword arguments:")
for key, value in kwargs.items():
print(f"{key}: {value}")
complex_function("Alice", 25, "Extra Arg 1", "Extra Arg 2", location="New York", occupation="Engineer")
异常处理的高级技巧
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f"Caught an exception: {e}")
except Exception as e:
print(f"Caught a more general exception: {e}")
finally:
print("This will always be executed")
装饰器的应用
def my_decorator(func):
def wrapper(*args, **kwargs):
print("Before function execution")
result = func(*args, **kwargs)
print("After function execution")
return result
return wrapper
@my_decorator
def sample_function(name):
print(f"Hello, {name}!")
sample_function("Bob")
上下文管理器
class MyContextManager:
def __enter__(self):
print("Entering the context")
return self
def __exit__(self, exc_type, exc_val, exc_tb):
print("Exiting the context")
if exc_type is not None:
print(f"Exception occurred: {exc_type}, {exc_val}")
return False
with MyContextManager() as cm:
print("Inside the context")
raise ValueError("This is an example exception")
并发与并行编程
import concurrent.futures
def long_running_task(name, duration):
print(f"Starting {name} for {duration} seconds")
import time
time.sleep(duration)
print(f"{name} completed")
with concurrent.futures.ThreadPoolExecutor(max_workers=2) as executor:
futures = [executor.submit(long_running_task, "Task 1", 3), executor.submit(long_running_task, "Task 2", 5)]
for future in concurrent.futures.as_completed(futures):
pass